Lab 8
Working from Home
If you are working from home, you must download the new jar
file named Classlibs.jar and place
it in your
c:\projects\CSE115\Classlibs\Spring2006\
folder.
Introduction
As the legend goes, Adrienne, Mike, Clark, and Ben were just hanging out
in a bar one Friday afternoon when who walks in, but Blinky, Inky, Pinky,
and Clyde (friends of Clark's - of course). They sashay up to their table
in their usual smarmy way and strike up a conversation with Clark, complete
with a secret handshake that only members of their particular fraternal organization
know. Who knew that Blinky, Inky, Pinky, and Clyde even had hands?
Anyway, Clark invites these well-known guests to join us and the conversation
quickly turns ugly. It was really all Pinky's fault. He started in with us
about the fact that OO had no place in their world. They had survived for
26 years without it and he even claimed that no one could even use OO to
create their world! Blinky agreed and added that NGP was not up to the challenge.
Inky threw in the last insult that there wasn't anyone in 115 this semester
with the programming chops to complete the task. Clyde just said "uh-huh"
and "yeah" a lot.
Needless to say, this enraged the 115 team and suddenly Mike jumps up to
separate Adrienne and Inky (whom Adrienne had in a head-lock), and Clark
pulls Blinky and Pinky away from the fray. What no one noticed is that Ben
had snuck under the table (to hide) and suddenly emerges with his laptop
and a completed implementation of the world using NGP and his OO skills.
This did not satisfy the boys who insisted that of course the four of us
could do it - we can do anything, bouncing balls, dizzy fish, etc. They wanted
to see the 115 students step up to the plate.
The negotiations began and a set of ground rules was established with
an agreement that the eight of them would meet again after May 1st with the
results of the challenge. Now it's up to you, the 115 students to assure
that the 115 staff doesn't have to foot the bill for a night of drinking
with those guys. Clyde downed 10 beers and 8 shots in the 30 minutes he was
at the bar the first time. Who knows what a whole night will bring? Don't
let us (and our wallets) down.
New Concepts Covered
The following are the new concepts covered in this lab.
- Collections of objects
- primitive types (boolean, int)
- control structures
if
statement
for
and while
loop
- Design Patterns
- State pattern
- Iterator pattern
Hints/Advice
The new concepts will be covered in lecture and in recitation. If you do
not attend both lecture and recitation, you will find this lab significantly
more challenging. Lecture material will be presented in the context of this
lab, or in a similar and related context. It is imperative that you start
this lab right away. This is, by far, the most challenging lab of the semester,
and it will be figured accordingly into your final grade. The instructors
and TAs will be happy to help those who heed this advice, but will grow short-tempered
with those who seek beginner's help just prior to the due date.
Lab Tasks
Create a new project in Eclipse
As with Lab 7, you should create your own project and name the project Lab8
and the package inside it pacman. Refer to the Lab 7 directions if you do
not remember how to do this.
Assignment Specification
So, if you didn't know, Blinky, Pinky, Inky and Clyde are the ghosts made
famous by the game Pac-Man. Your assignment is to write a somewhat simplified
version of PacMan. If you have not ever seen or played the game Pac-Man,
you can refer to a Wikipedia article on the subject, or google Pac-Man
to find a version you can play online or even download for your cell phone.
In this assignment, you will be able to complete basic functionality for
a possible score of 100, or complete basic functionality plus extra credit
for a possible score greater than 100. It is most important that you complete
the basic functionality before attempting the extras. Note that, even in
the Submission Directions, you must submit
a basic game separate from the game with extras.
Basic requirements (100 points)
The basic requirements are:
- For a grade of F [48 points] Basic gameboard requirements:
- A game window appears at start up that does not have
the board configuration showing[2 points], but does provide:
- [2 points] A way for the user to start the game
- [2 points] Directions about how the user should move the
Pac-Man
- [2 points] Directions about how the user can pause the game
- [2 points] The ability to end the game (quit functionality)
- When the user presses start:
- [10 points] The game board should
appear with walls, dots, power pellets, and passageways present
(looking like the traditional Pac-Man board)
- [5 points] Pac-Man appears on the screen
- [5 points] The four ghosts appear on the screen (in the ghost cage)
- [5 points] Pac-Man should be moving when the game begins
- A pause/unpause game functionality should be implemented
so that when a user selects to pause the game, all of the animated
elements
stop moving [3 points] and the Pac-Man, dots, power pellets, and ghosts disappear [5 points]
from the screen. Unpausing the game resumes normal game functionality [5 points].
- For a grade of D [54 points] Implement all of the above plus:
- [6 points] Pac-Man stops moving when he hits a wall.
-
For a grade of C+ [64 points] Implements all of the above
plus:
-
For a grade of B- [73 points] Implement all of the above
plus:
-
For a grade of B [79 points] Implement all of the above
plus:
-
Ghosts move around the screen as well. Ghosts can
not go through walls and therefore must move on the same pathways
as Pac-Man. Ghosts can not move diagonally, but they can utilize
the passageways. For the basic game functionality ghosts do not have
to actively seek out Pac-Man, they simply need to move around the
screen while the game is running.
-
For a grade of B+[84 points] Implement all of the above
plus:
-
[3 points] If Pac-Man encounters a ghost on his journey, he
dies
-
[2 points] If Pac-Man dies three times, the game is over and
the user must begin again.
-
For a grade of A-[89 points] Implement all of the above
plus:
-
[2 points] When Pac-Man encounters a dot on his journey, he eats it and the dot is removed
from the screen.
-
[1 point] Eating a dot causes Pac-Mans score to go up by 10 points.
-
[2 points] If Pac-Man eats all of the dots on the board, a new board appears.
-
For a grade of A[100 points] Implement all of the above
plus:
-
[2 points] When Pac-Man encounters a power pellet on his journey, he eats it and the dot is
removed
from the screen, his score goes up by 50 points, and he becomes Super Pac-Man.
-
[2 points] When Pac-Man becomes Super Pac-Man ghosts enter a vulnerable state and change
appearance on the screen.
-
[2 points] If Pac-Man encounters a ghost in its vulnerable state, Pac-Man eats the ghost and
his score goes up by 200 (for the first ghost), 400 if he eats 2 ghosts, 800 if he eats three ghosts, and 1600 if he eats
all four ghosts.
-
[3 points] When a ghost has been eaten, it disappears off the screen and reappears in a
non-vulnerable state in the ghost cage.
-
[2 points] The Super Pac-Man behavior wears off after a certain amount of time.
- You must submit a UML diagram with your solution to Lab 8. [If not submitted,
you will lose 10 points]
- Prepare a plain-text README file describing: [If
not submitted, you will lose 10 points]
- the testing of your code that you have done, and
- all known bugs with your code. This is important - if we find
bugs that you haven't listed, we will deduct extra points. If we
find bugs you have listed, then we will not deduct additional points
for those bugs.
Extensions (215 points extra credit described
so far - may be adding more!)
The following are possible extensions that you may choose to undertake.
If you have an idea that is not on the list, send email to your instructor
for approval of it as an extension and a point breakdown.
- [10 points] More intelligent ghost behavior. Have the
ghosts implement some sort of smarts to chase after Pac-Man, and run
away from him when he is Super Pac-Man.
- [10 points] Implement actual Pac-Man behaviors for
each one of the four ghosts. You can find an explanation of their original
behaviors on many sites (even the Wikipedia). You will not earn credit
for both this and the option above.
- [10 points] Implement the "fruits" that would randomly
appear on the board for a certain amount of time and would allow Pac-Man
to earn extra points.
- [10 points] Two-player option that alternates players
after each player loses a life (the traditional two-player Pac-Man).
- [20 points] Two-player option where both players are playing
on the screen at the same time on two different boards.
- [20 points] Two-player option where both players are playing
on the same board on the same screen and whomever gets the most points
on that board wins.
- [20 points] Two-player option where more adversarily
confrontation, player one is regular Pac-Man and player two is "evil"
Pac-Man, ignoring the dots and power pellets and only trying to eat
the other Pac-Man.
- [20 points] Be able to play the game as Pac-Man or as
a ghost, where the ghosts earn points for "getting" Pac-Man.
- [25 points] Multiple player option where each player can
be a ghost or the Pac-Man and the ghosts can attack each other as well
as Pac-Man - this means up to five players could play the game simultaneously.
- [15 points] Each level of the game displays a different
board.
- [10 points] Rewrite the board creation class so that it
creates a different board configuration. For these points, each level
of the game can have the same board, but it must be significantly different
than the original board.
- [3 points] Add sounds to the game.
- [7 points] Add better graphics to the game (ie - a Pac-Man
that is animated with the traditional munching action)
- [10 points] Pacman is an image of the student's head, and
the four ghosts are images of Ben, Mike, Clark, and Adrienne's heads, with
eating animation fully intact.
- [4 points] Implement some sort of high score where you can
enter a name and it shows the top few high scores. Note: does not have to
maintain this information when the program is closed.
- [6 points] Change the high score so that it saves the high
scores to a file or the like. This way we can save the high scores even
when the program is closed.
- [15 points] Modify the walls so that they look exactly
like the original pacman board. The walls are thin blue lines and they curve
appropriately around corners. See image:
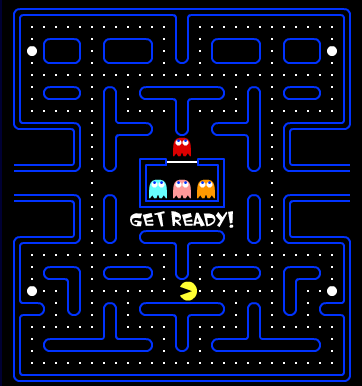
Submission Directions
You must include in the project a README file, as indicated above.
This file must be a plain text file. You can create a plain text file in
Eclipse by right clicking on your project and selecting New -> Untitled
Text File. Now select File->Save As. Navigate to the Lab8 package within
your Lab8 project (you should see "Lab8/lab8" as the parent folder
location). Name the file "README" and click OK.
After you are finished writing your code, confirm that your .java files
and the README file are located in the Lab8 package in your Lab8
project. Once you are certain that you have the correct set of files in your
project, export your solution as a jar file, just as you did in previous
labs, but name your jar file Lab8.jar
.
VERY IMPORTANT NOTE- If you decide to do work beyond the minimum requirements,
you must do the following:
- A submission with just your basic requirements, named Lab8.jar.
- A separate submission with the extra work included, named Lab8Extra.jar.
- The Lab8Extra.jar file must be able to
be imported as an exisiting project entitled "Lab8Extra." This means that the project
folder name must be "Lab8Extra" before you attempt
to export.
- In your Lab8Extra README file, you must include a list of the
extra credit items you completed, as well as the testing and bug
information for the extra credit items.
You must follow all directions about filenames
exactly, otherwise your work will not be graded.
When you are ready to submit your work, use the electronic
submission program that corresponds to your recitation.
Due Dates
Due 11:59:59pm on Monday May1st for all sections.