|
The picture on the left includes three relationships:
Local Variable Dependence Relationship
Composition Relationship
Association Relationship
(Mutator Methods)
(Accessor Methods)
This example is also part of the lab4 assignment, MainClass.java which needs to be created by ourselves in the lab4.
I simplified the MainClass.java and only added one button to the Window so that the relationship will be clearer to see. |
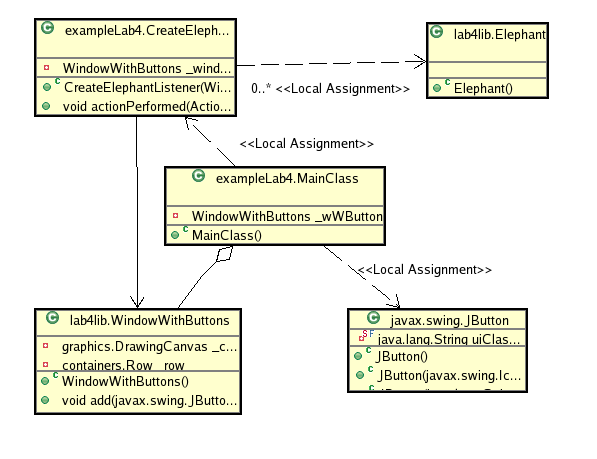 |
// code for the UML of MainClass.java showed above
package exampleLab4;
nnnimport javax.swing.JButton;
nnnimport lab4lib.WindowWithButtons;
public class MainClass {
nnnnnnnnnnnprivate WindowWithButtons _wWButton;
public MainClass() {
nn_wWButton=new WindowWithButtons();
nnJButton button1=new JButton("Create elephant");
nn_wWButton.add(button1);
nnCreateElephantListener cIL= new CreateElephantListener(_wWButton);
nnbutton1.addActionListener(cIL);
}
}
|
|
Local Variable Dependency Relationship and Instantiation Dependency Relationship
Local Variable Dependency Relationship is the relationship between two class. Informally, it is called the "use a" relationship. In the example, the relationship between exampleLab4.MainClass and example.Lab4.CreateElephantListener,exampleLab4.MainClass and javax.swing.JButton are Local Variable Dependency Relationship.
In the UML diagram, two classes that are in the instantiantion dependency relation ship have a dashed arc with an arrow head between them. The arrow points from the "user" class to the class it is using.
In the example, the class "exampleLab4.MainClass" is using the class "example.Lab4.CreateElephantListener" in its .java file.
In the code,
CreateElephantListener cIL= new CreateElephantListener(_wWButton);
(relationship between exampleLab4.MainClass and example.Lab4.CreateElephantListener)
JButton button1=new JButton("Create elephant");
(relationship between exampleLab4.MainClass and javax.swing.JButton)
Instantiation Dependency Relationship is similar as Local Variable Dependency Relationship. Instead of using a Local Variable, it creates an object of the class that it is using directly. For example if we write the code as
new CreateElephantListener(_wWButton);
then it is an Instantiation Dependency Relationship between exampleLab4.MainClass and example.Lab4.CreateElephantListener. In this case, we did not explicitly declare a variable for the reference, but the reference is stored in the memory as well. This kind of variable that doesn't have a name is called an anonymous variable. In the UML, the diagram is similar as in the Local Variable Dependency Relationship and the only difference is that <<Instantiation>> appears in the place where <<Local Assignment>> is.
back to top |
Composition Relationship
Composition relationship is also called a whole-part relationship. It models the relationship between a whole and one of its integral parts. Usually, the "part object" is the description of the property of the "whole object." Informally, it is referred to as the "has a" relationship. It involves the use of Instantiation variable and the lifetimes of the objects involved in this relationship are intimately tied together.
In the example, the relationship between exampleLab4.MainClass and the lab4lib.WindowWithButtons are such kind of relationship.
In the UML diagram, it is shown as a solid line connect between the two classes involved in the relationship. The line is marked with a diamond shape at the "whole" class. In the example, the exampleLab4.MainClass is the whole part and the lab4lib.WindowWithButtons is its integral part.
In the code, it is shown as the orange part. The "part object" is declared within the class of the "whole object"and outside the methods and the relationship is created within the constructor of the "whole" part.
public class MainClass {
private WindowWithButtons _wWButton;
public MainClass() {
_wWButton=new WindowWithButtons();
JButton button1=new JButton("Create elephant");
_wWButton.add(button1);
CreateElephantListener cIL= new CreateElephantListener(_wWButton);
button1.addActionListener(cIL);
}
}
back to top |
Association relationship
Association relationship can be described as a relationship between the "source part" and the "target part."There are some similarity between the composition relationship and this one, which involves the using of the instantiation variable and enables communication between two object. However, the lifetimes of objects involved in the association relationship do not tie to each other. In other words, the "target object" is changable overtime with no affection to the existence of the "source part."
In the example, the relationship between example.Lab4.CreateElephantListener and lab4lib.WindowWithButtons are such kind of relationshiip.
In the UML diagram, it is shown as a solid line between the source class and the target class, with an open arrowhead pointing to the target class. In the example, the example.Lab4.CreateElephantListener is the source part and the lab4lib.WindowWithButtons is the target part.
The code is in the file CreateElephantListener.java shown in the home page as the blue part. The source class has a reference to an instance of the target class. Thus, there is an assignment to the instance variable declared to be the target class' type. We can also use the "Mutator Methods" to establish an association relationship.
public class CreateElephantListener implements ActionListener {
private WindowWithButtons _windowWithButtons;
public CreateElephantListener(WindowWithButtons windowWithButtons) {
_windowWithButtons = windowWithButtons;
}
public void actionPerformed(ActionEvent ag0) {
Elephant elephant;
elephant = new Elephant();
_windowWithButtons.add(elephant);
}
}
back to top |
Mutator Methods
If we want the target to be changable overtime, we can include a method which we can call to assign a new value to the instance variable instead of passing the value to the constructor. It is also called a "setter" method, because it sets the value of an instance variable. In the example, we can make changes like this,
public void setWindow(WindowWithButton windowWithButton){
_windowWithButtons = windowWithButton;
}
In this case, the code for the can be written as:
public class CreateElephantListener implements ActionListener {
private WindowWithButtons _windowWithButtons;
public CreateElephantListener() {
}
public void setWindow(WindowWithButtons windowWithButtons){
_windowWithButtons = windowWithButtons;
}
public void actionPerformed(ActionEvent ag0) {
Elephant elephant;
elephant = new Elephant();
_windowWithButtons.add(elephant);
}
}
Or we can combine the two approaches together so as to require that the initial balue of the variable be specified via the constructor, yet still permit its value to be changed via the mutator:
public class CreateElephantListener implements ActionListener {
private WindowWithButtons _windowWithButtons;
public CreateElephantListener(WindowWithButtons windowWithButtons) {
_windowWithButtons = windowWithButton;
}
public void setWindow(WindowWithButtons windowWithButton){
_windowWithButtons = windowWithButton;
}
public void actionPerformed(ActionEvent ag0) {
Elephant elephant;
elephant = new Elephant();
_windowWithButtons.add(elephant);
}
}
back to top |
Accessor Methods
Another method that involves the using of instantiation relationship is the Accessor Methods, whose job is to provide the current value of an instance variable to the caller of the method. It is also called a "getter method", because it gets the value of an instance variable.
According to the example of CreateElephantListener.java, the accessor method can be written as: public WindowWithButtons getWindow(){
return _windowWithButtons;
}
back to top |
|